Development Blog
Facade Pattern
The primary goal of a Facade Pattern is not to avoid you having to read the manual of a complex API. It's only a side-effect. The first goal is to reduce coupling and follow the Law of Demeter.
A Facade is meant to decouple a client and a sub-system by embedding many (but sometimes just one) interface, and of course to reduce complexity.
A facade does not forbid you the access to the sub-system
You can (you should) have multiple facades for one sub-system
That's why a good facade has no new in it. If there are multiple creations for each method, it is not a Facade, it's a Builder or a [Abstract|Static|Simple] Factory [Method].
The best facade has no new and a constructor with interface-type-hinted parameters. If you need creation of new instances, use a Factory as argument.
The above may sound complicated but essentially all a Facade is doing is grouping code together so if you need to call it, it's simple and easier to understand whats going on, i.e:
Adding this to code:
$this->eatBreakfast();
Beats this (this would be encapsulated inside eatBreakfast()):
$this->openFridge();
$this->getSausages();
$this->getEggs();
$this->getBacon();
$this->cook();
$this->openMouth();
$this->swallow();
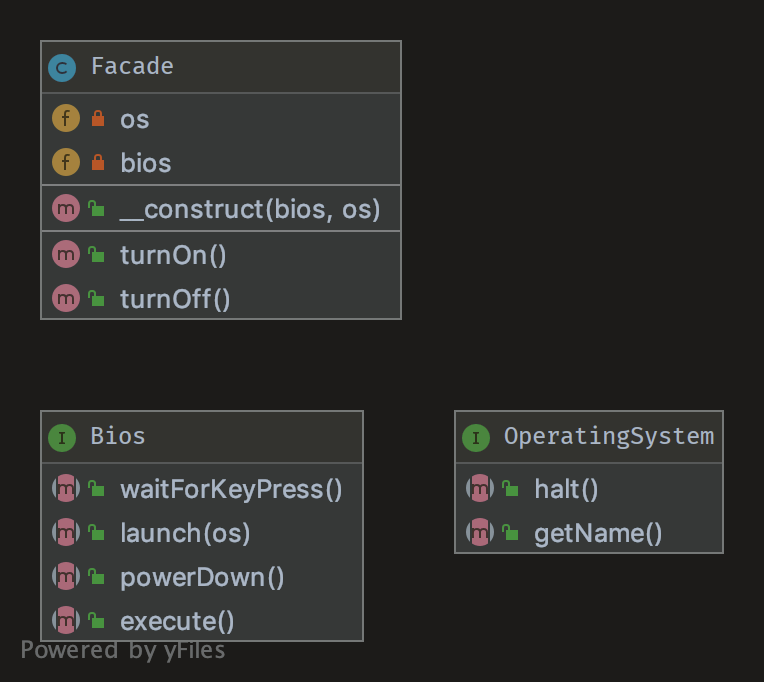
Bios
namespace DesignPatterns\Structural\Facade;
interface Bios
{
public function execute();
public function waitForKeyPress();
public function launch(OperatingSystem $os);
public function powerDown();
}
Operating System
namespace DesignPatterns\Structural\Facade;
interface OperatingSystem
{
public function halt();
public function getName(): string;
}
Facade
namespace DesignPatterns\Structural\Facade;
class Facade
{
public function __construct(private Bios $bios, private OperatingSystem $os)
{
}
public function turnOn()
{
$this->bios->execute();
$this->bios->waitForKeyPress();
$this->bios->launch($this->os);
}
public function turnOff()
{
$this->os->halt();
$this->bios->powerDown();
}
}